As a full-stack web developer I spend a lot of time switching between back-end and front-end code. When you’ve been working in TypeScript or JavaScript ES6, coming back to PHP and it’s lack of short form for anonymous functions and closures is a real frustration.

TypeScript closure
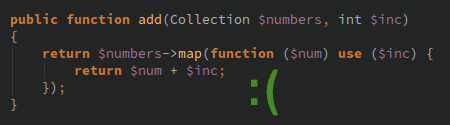
PHP Closure
Whilst we all continue to wait for the progress of the PHP RFC for arrow functions, fortunately there are some tricks you can use to make it a lot less painful for creating these functions in PHP.
Using Live Templates
Keyboard macros or expansions are a simple productivity trick that I think every developer should make use of. These allow you to type only a few characters and then use a key combination (usually tab) to expand this to a much longer output that saves you typing it out manually.
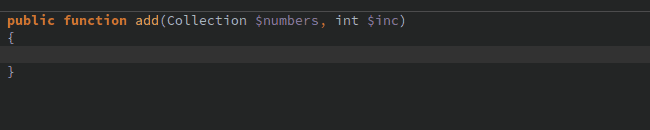
Live Templates in action in PHPStorm
Most modern IDEs support these shortcuts. I use PHPStorm and these are known as Live Templates. I have setup a few Live Templates to make it easier to create these PHP functions, mirroring the JavaScript syntax as closely as possible. PHP is pretty unique in having the =>
syntax for associative arrays, so unfortunately we can’t just expand that directly! I elected to use the shortcuts f=>
for a simple anonymous function, and f=>u
where we need a closure that shares one or more variables from the current scope.
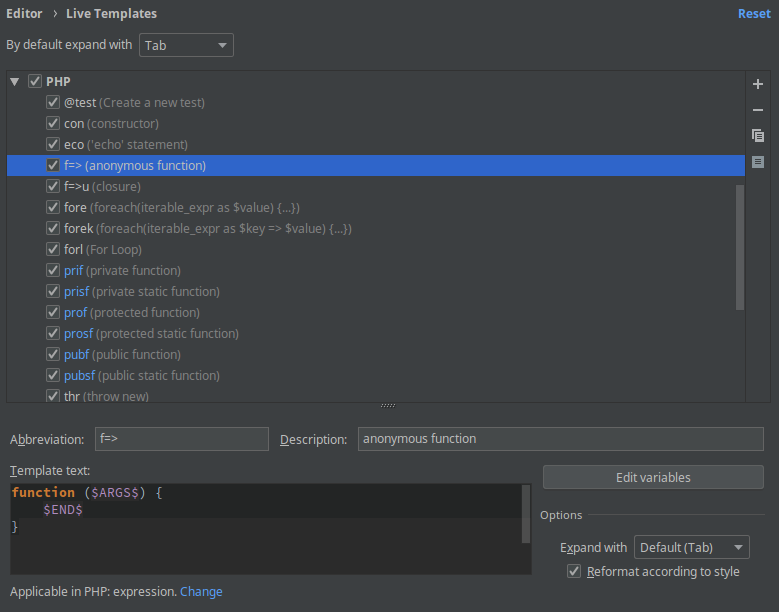
Live Templates for JavaScript-like functions in PHPStorm
This means we can then just type f=>
and then press tab
and the IDE will auto-complete the rest of the syntax for the function. Even better, hitting tab will move the cursor directly from the function arguments straight to the function body so we can start typing right away.
Importing Live Templates
If you are also a PHPStorm user, you can easily import these Live Templates into your editor. Just copy the XML code below, then go into the Live Templates area in the IDE settings, right-click one of the appropriate groups (PHP would make sense!) and hit paste.
<template name="f=>" value="function ($ARGS$) { $END$ }" description="anonymous function" toReformat="true" toShortenFQNames="true">
<variable name="ARGS" expression="phpSuggestVariableName()" defaultValue="" alwaysStopAt="true" />
<context>
<option name="PHP Expression" value="true" />
</context>
</template>
<template name="f=>u" value="function ($ARGS$) use ($USES$) { $END$ }" description="closure" toReformat="true" toShortenFQNames="true">
<variable name="ARGS" expression="phpSuggestVariableName()" defaultValue="" alwaysStopAt="true" />
<variable name="USES" expression="phpVar" defaultValue="" alwaysStopAt="true" />
<context>
<option name="PHP Expression" value="true" />
<option name="PHP Statement" value="true" />
</context>
</template>
If you don’t like the shortcuts I’ve chosen you can easily customise them to something that suits you.
Advanced Live Templates
Live Templates can get a lot more advanced and can really start to save you time if you repeat a lot of similar code constructs during your development. See below for another example I use a lot for creating a new instance of a model from a Faker factory for testing, complete with PHPDoc for IDE completion hints.
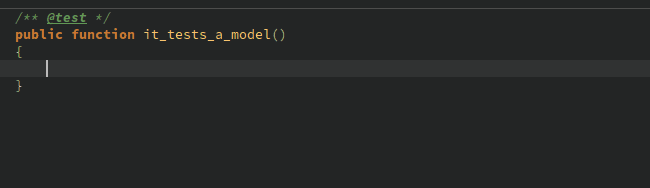
Live Template for creating a new Model from a Faker factory
Here is the XML code to copy for importing the above example into PHPStorm:
<template name="tmodel" value="/** @var $CLASS$ $$$VAR_NAME$ */ $$$VAR_NAME$ = static::$fm->create($CLASS$::class); $END$" description="Create test instance of Model" toReformat="true" toShortenFQNames="true">
<variable name="CLASS" expression="classNameComplete()" defaultValue="" alwaysStopAt="true" />
<variable name="VAR_NAME" expression="decapitalize(CLASS)" defaultValue="" alwaysStopAt="false" />
<context>
<option name="PHP" value="true" />
</context>
</template>
Hopefully you found this post useful and it inspires you to create a few Live Templates or other shortcuts of your own to increase your productivity.